Open, enterprise-grade licensing & distribution
Keygen is a fair source software licensing and distribution API, built for developers, by developers. Self-host or try Keygen Cloud today.
Free during development, no upfront commitment
Developer-first
We've designed Keygen's API to be easy to use for developers of all skill sets. Use our official SDKs or grab your favorite HTTP library.
keygen.LicenseKey = "C1B6DE-39A6E3-DE1529-8559A0-4AF593-V3" // Validate the licenselicense, err := keygen.Validate(fingerprint)if err == keygen.ErrLicenseExpired { panic("license is expired!")} if err == keygen.ErrLicenseNotActivated { // Activate the license machine, _ := license.Activate(fingerprint) fmt.Printf( "activated machine %s for license %s!\n", machine.ID, license.ID, )}
Cloud-native
Keygen was born in the cloud. Cloud is in our team's DNA. With Keygen Cloud, we'll handle all the hard stuff while your team focuses on product.
Focus
Let your team focus on product, not in-house licensing and distribution. Save time by using Keygen Cloud as a ready-to-go licensing solution.
Maintenance
Skip the expensive up-front and ongoing costs of an in-house or self-hosted licensing solution. Save your business development time and money with Keygen Cloud.
Scale
Effortlessly go from hundreds of users, to thousands, to hundreds of thousands and beyond. We'll handle the infrastructure so your team can focus on product.
Security
We strive to ensure that data stored within Keygen Cloud is as secure as possible. TLS, HA, continuous backups, data encryption at-rest, in-transit, and at-work.
Enterprise-grade
Keygen EE, our Enterprise Edition, is perfect for businesses that want to self-host Keygen.
Keygen EE comes with the following:
- Historical request logs
- Event/audit logs
- Environments
- Permissions
- Self-hosting support
- SAML/SSO*
* SAML/SSO is under development.
docker run --name keygen.1 -p 3000:3000 \ -e REDIS_URL="redis://host.docker.internal:6379" \ -e KEYGEN_LICENSE_FILE_PATH="/etc/keygen/ee.lic" \ -e KEYGEN_LICENSE_KEY="C1B6DE-39A6E3-DE1529-8559A0-4AF593-V3" \ -e KEYGEN_ACCOUNT_ID="c8ef4b4b-9a0a-49c7-8ea4-c47d13aa11f9" \ -e KEYGEN_HOST="licensing.enterprise.example" \ -e KEYGEN_MODE="singleplayer" \ -e KEYGEN_EDITION="EE" \ -v /etc/keygen:/etc/keygen \ keygen/api web
Fair-source
Keygen CE, our Community Edition, is free to self-host for personal and commercial projects.
Free
Keygen CE is free (as in beer) to self-host for any project. We want to make licensing accessible for everyone.
Open
Keygen is licensed under the Fair Core License. Browse the code on GitHub, clone it, fork it, modify it, use it.
Community
Join our communities on GitHub and Discord to make friends, talk shop, and get community support.
Contribute
Want to see something new in Keygen? Open an issue or pull request and let's talk about your new idea.
Ready, set, run
Clone it, run the setup, then serve. That's it. Self-hosting made easy.
git clone https://github.com/keygen-sh/keygen-api && cd keygen-apibundlebundle exec rails keygen:setupbundle exec rails server
Trusted by over 6,000 software businesses all over the worldincluding these industry leaders
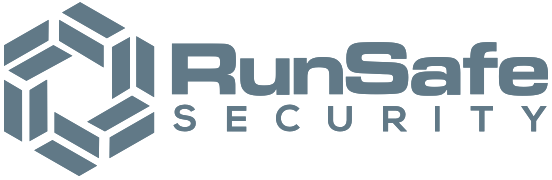
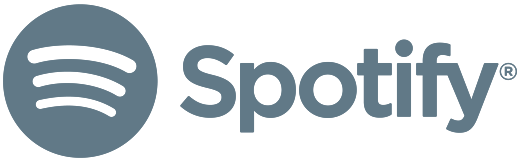

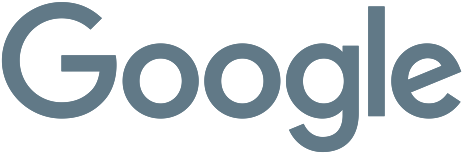
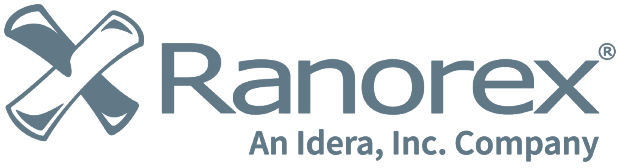
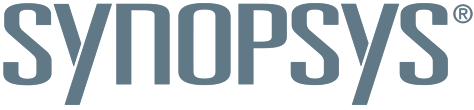
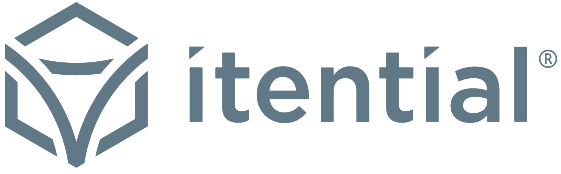
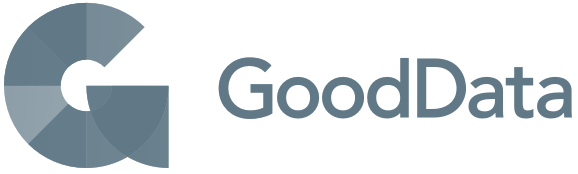
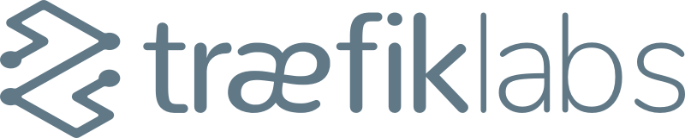