Ready, set, ship
From licensing to automatic updates, we've got you covered. Dive in with Keygen Cloud, or explore our self-hosted options.
- Utilize our software distribution API to allow downloads for licensed users. Integrate directly with industry-standard tooling like Squirrel or NSIS for painless automatic updates.
- Protect your app with our flagship software licensing API. Add powerful entitlement constraints, upgrade version constraints, enforce activation limits, and more.
using System.Threading.Tasks;using Squirrel; static async Task Main(){ var licenseToken = "lic-6171564a4a59e5f3879c0584ebe92ce3v3"; var releases = $"https://get.keygen.sh/demo/win32?auth=token:{licenseToken}"; using (var mgr = new UpdateManager(releases)) { await mgr.UpdateApp(); }}
Trusted by over 6,000 software businesses all over the worldincluding F1000s, AI startups, SMBs, and indies
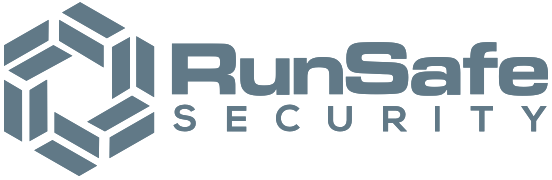
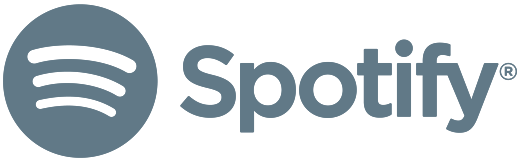

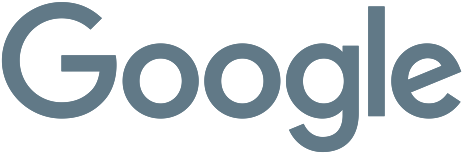
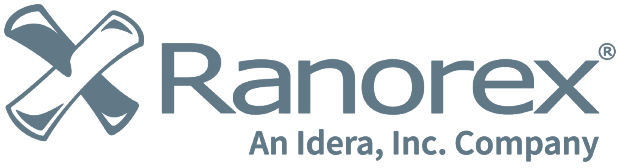
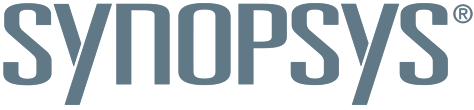
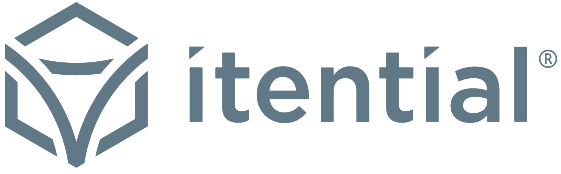
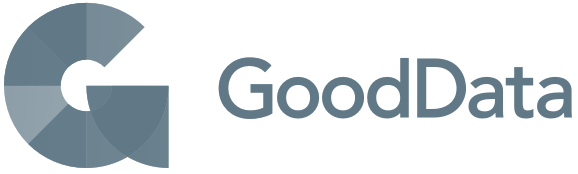
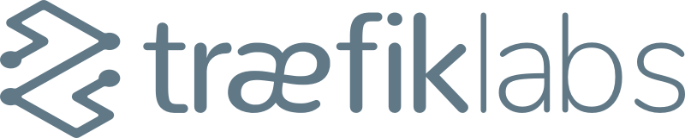
Solutions for licensing & distribution
dns Choice
Managed Keygen Cloud, or explore self-hosting.
library_books Quickstarts
Guides and API references for developers of all skill levels.
insert_chart Dashboard
Manage your products from an intuitive dashboard.
business Pricing
From indie to enterprise, we have options for everyone.