Ready, set, ship
From licensing on-prem to multi-prem, we've got you covered. Dive in with Keygen Cloud, or explore our self-hosted options.
- Protect your IP using our flagship software licensing API. Add fine-grained entitlement constraints, enforce node- and CPU-based maximums, add metering, and more.
- Use our software distribution API as a private container store to securely deliver images and other binary artifacts to licensees.
export KEYGEN_LICENSE_KEY='C1B6DE-39A6E3-DE1529-8559A0-4AF593-V3' docker build \ "https://license:${KEYGEN_LICENSE_KEY}@get.keygen.sh/demo/docker/keygen.dockercontext"# => Uploading context 10240 bytes# => Step 1/3 : FROM keygen# => Pulling repository keygen# => ---> e9aa60c60128MB/2.284 MB (100%) endpoint: https://get.keygen.sh/# => Step 2/3 : RUN ls -lh /# => ---> Running in 9c9e81692ae9# => total 24# => drwxr-xr-x 2 root root 4.0K Mar 12 2013 bin# => drwxr-xr-x 2 root root 4.0K Oct 19 00:19 etc# => drwxr-xr-x 2 root root 4.0K Nov 15 23:34 usr# => ---> b35f4035db3f# => Step 3/3 : CMD echo Hello world# => ---> Running in 02071fceb21b# => ---> f52f38b7823e# => Successfully built f52f38b7823e# => Removing intermediate container 9c9e81692ae9# => Removing intermediate container 02071fceb21b
Trusted by over 6,000 software businesses all over the worldincluding these industry leaders
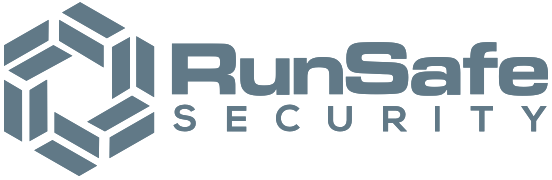
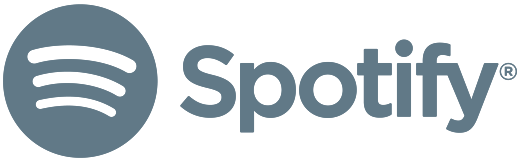

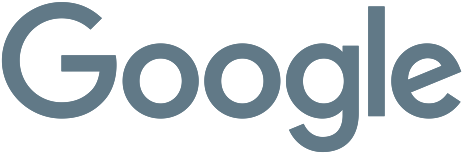
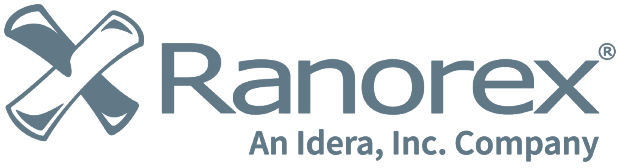
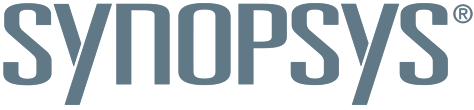
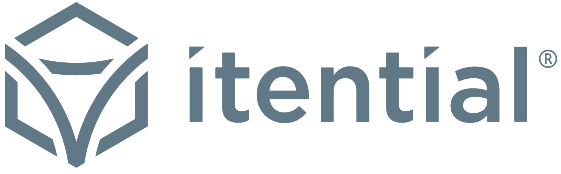
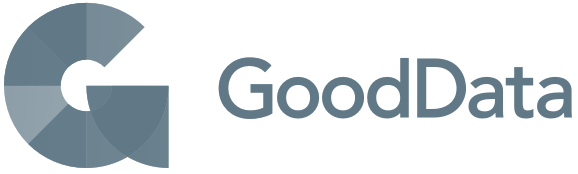
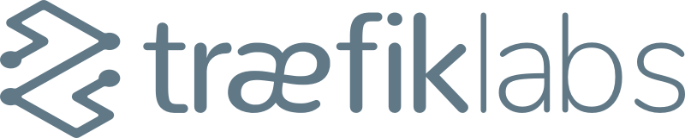
Solutions for licensing & distribution
dns Choice
Managed Keygen Cloud, or explore self-hosting.
library_books Quickstarts
Guides and API references for developers of all skill levels.
insert_chart Dashboard
Manage your products from an intuitive dashboard.
business Pricing
From indie to enterprise, we have options for everyone.